The regular expressions are useful in finding the patterns in the data. These operations are basically used to find and replace operations performed on the string. In Scala, a string is changed into a regular expressing by using the .r method. (.r) is denoted as Regex that is used for text parsing and present in scala. util.matching package.
The following is an example of a regular expression pattern in which we will use Regex(regular expression) to make sure a password contains a number. Download the program and run it from the Scala shell.
import scala.util.matching.Regex
val regularexp: Regex = "[0-9]".r
regularexp.findFirstMatchIn("awesomepassword") match {
case Some(_) => println("Password OK")
case None => println("Password should contain a number")
}
Output
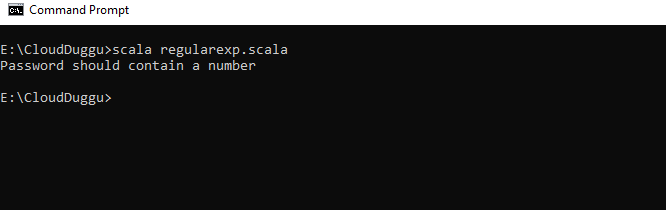
Click Here To Download Code File.
Scala Regular Expressions
The following is the list of regular expression operators that are supported in Scala.
Expression | Actions |
---|---|
^ | This expression matches the beginning of the line. |
$ | This expression matches the end of the line. |
. | It matches any of the single characters beside newline. |
[...] | This expression matches any single character in brackets. |
[^...] | This expression matches any single character, not in brackets. |
\\A | Beginning of the entire string. |
\\z | End of the entire string. |
\\Z | It represents the end of the entire string. |
re* | This expression matches 0 or more occurrences of the preceding expression. |
re+ | This expression matches 1 or more of the previous thing |
re? | It matches 0 or 1 event of the expression. |
re{ r} | This expression matches exactly r number of occurrences of the preceding expression. |
re{ y,} | This expression matches y or more occurrences of the preceding expression. |
re{ y, z} | This expression matches at least y and at most z occurrences of the preceding expression. |
a|b | This expression matches either a or b. |
(re) | This expression Groups regular expressions and remembers matched text. |
(?: re) | This expression Groups regular expressions without remembering matched text. |
(?> re) | This expression matches an independent pattern without backtracking. |
\\w | This expression matches word characters. |
\\W | This expression matches nonword characters. |
\\s | This expression matches whitespace. Equivalent to [\t\n\r\f]. |
\\S | This expression matches non-whitespace. |
\\d | This expression matches digits. Equivalent to [0-9]. |
\\D | This expression matches non-digits. |
\\A | This expression matches the beginning of the string. |
\\Z | This expression matches the end of the string. |
\\z | This expression matches the end of the string. |
\\G | It indicates the point where the last match was finished. |
\\n | Back-reference to capture group number "n" |
\\b | This expression matches word boundaries when outside brackets. Matches backspace (0x08) when inside brackets. |
\\B | This expression matches nonword boundaries. |
\\n, \\t, etc. | This expression matches newlines, carriage returns, tabs, etc. |
\\Q | This expression is used to quote all characters till \\E. |