The Update() method is the part of MongoDB CRUD operations that are used to update the values in the existing Documents of a Collection.
In this section of the Tutorial, we will go through the below list of MongoDB Update Methods.
- db.collection.updateOne()
- db.collection.updateMany()
- db.collection.replaceOne()
Let us see each MongoDB Update Method in detail with the example.
db.collection.updateOne()
The updateOne() method is used to update the single Document in the Collection.
Syntax:
The following is the syntax of the updateOne() method.
Let us see the example of updateOne() method. In the Insert Document section, we already inserted the following Documents in the item_inventory collection of the mongodb_test database.
[ { item_name: "journal", item_quantity: 25 , item_tags: ["blank", "red"], item_size: { height: 14, weight: 21, uom: "cm" } },
{ item_name: "mat", item_quantity: 85, item_tags: ["gray"], item_size: { height: 27.9, weight: 35.5, uom: "cm" } },
{ item_name: "laptop", item_quantity: 100, item_tags: ["black"], item_size: { height: 10, weight: 2, uom: "cm" } },
{ item_name: "mouse", item_quantity: 110, item_tags: ["white"], item_size: { height: 3, weight: 4, uom: "cm" } },
{ item_name: "mousepad", item_quantity: 25, item_tags: ["gel", "blue"], item_size: { height: 19, weight: 22.85, uom: "cm" } }
] )
Now we will update the "item_name: "journal"" and use the $set to set the value of "item_quantity : 30". Once the command is executed we will verify if the value is changed using the find() method.
Example:
{ item_name: "journal" },
{
$set: { item_quantity : 30 }
}
)
Output:
We can see from the below output that the value of "item_quantity" is changed to "30".
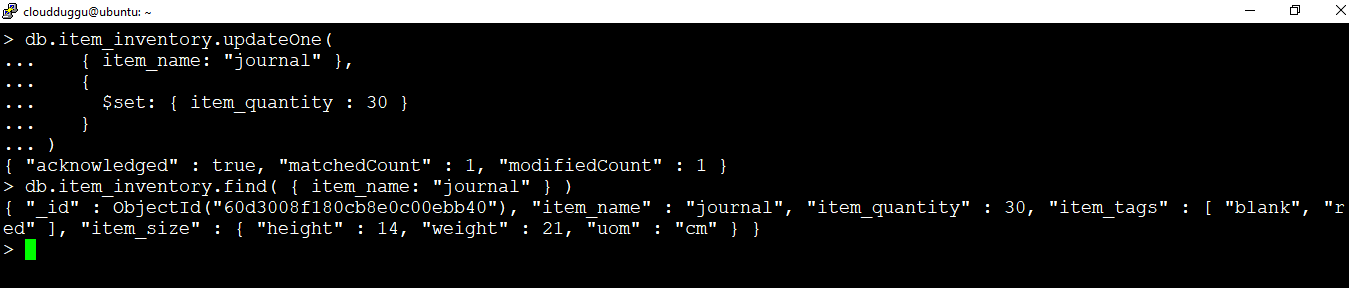
db.collection.updateMany()
The updateMany() method is used to update all documents that match the filter condition.
Syntax:
The following is the syntax of updateMany() method.
In the below example we will update "item_size.uom": "in" of the Document where the "item_quantity" is less than($lt) 50.
Example:
{ "item_quantity": { $lt: 50 } },
{
$set: { "item_size.uom": "in"}
}
)
Output:
We can see the 2 match counts were found and the records are updated. We can verify this using the find() method.
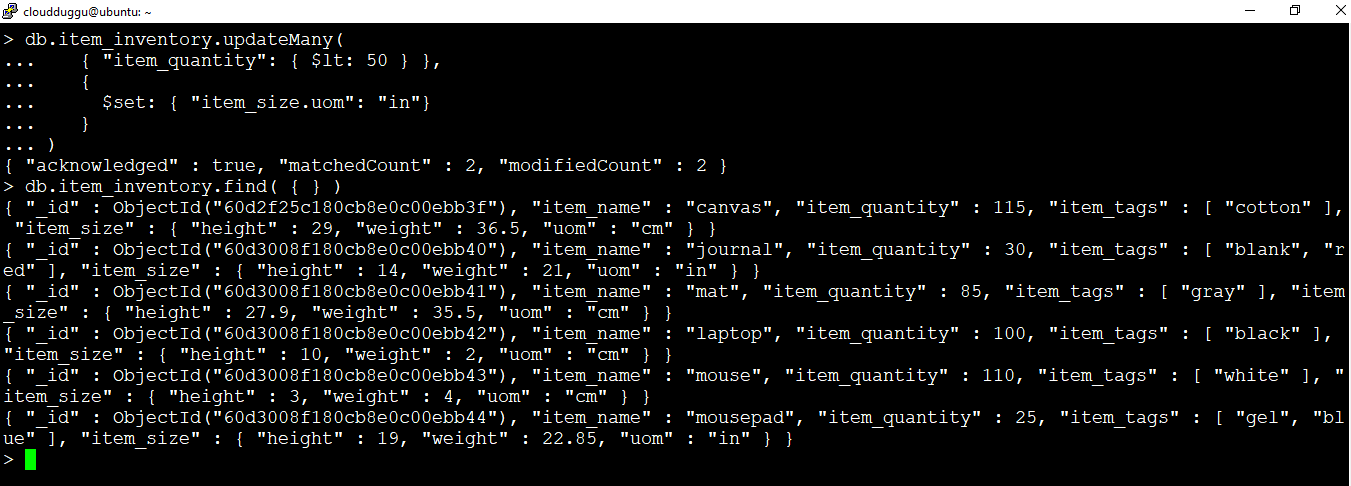
db.collection.replaceOne()
The replaceOne() command is used to replace a single document based on the matching filter condition.
Syntax:
The following is the syntax of replaceOne() method.
In this example, we will replace the Document value of "item_name: "laptop" with the below datasets.
Example:
{ item_name: "laptop" },
{ item_name: "laptop", item_size: [ { height: 10, weight: 2, uom: "cm" }, { height: 20, weight: 5, uom: "im" } ] }
)
Output:
From the below output we can see the dataset "item_name: "laptop" has been replaced.
